Solana Dev 101 - Creating a SvelteKit App with the New DAS API
Introduction
The Digital Asset Standard API (DAS) SDK simplifies the magic of compression and DAS on Solana by introducing types, one-liner abstractions, and properly formatted responses.
This step-by-step tutorial will guide you on using the DAS SDK to display NFT collection images on a web page using the getAssetsByGroup method. Moreover, this tutorial will lay the foundation for using the DAS SDK in more complex scenarios.

Prerequisites
To get the most out of this tutorial, make sure you have the following prerequisites:
- npm or yarn installed: These are package managers which are necessary to manage the project's dependencies.
- Basic knowledge of JavaScript: You should be comfortable with JavaScript fundamentals like variables, functions, and objects.
- Git installed: This is required for version control and collaboration.
Setting up your environment
To follow along with this tutorial, we recommend using the provided boilerplate repository, located here.
Check out a live view of this build, here.
Step 1: Setup a new SvelteKit project
First, we will need to set up a new SvelteKit project. You can use the create-svelte template to set up the project structure. Run the following command in your terminal:
Then navigate to the new directory:
Next, install the project dependencies:
Step 2: Set up the utility function
In the src/lib/util directory that is set up, create a new file named collection.ts.
Inside this file, set up a getCollection function, as shown below:
The getCollection function is an asynchronous function that will retrieve a collection of NFT data from a DAS API. It uses the Helius SDK to make RPC requests instead of having to set up a fetch or axios call.
- Begin the function by checking if there's any data stored in the local storage under the key 'nftData'. If there is, parse that JSON data and return it.
This is a form of caching to reduce unnecessary network requests if the data has already been fetched previously. You can input your own custom cache and loading logic.
2. Next, we initiate our Helius client with our API key. You can optionally pass in what cluster you’d like to be on, as well as your request id for the RPC.
- We initialize a couple of variables for pagination. We'll use these to paginate through the results from the DAS API, fetching 1000 items at a time until we've fetched all items.
- We enter a while loop that will continue running as long as paginate is true. Inside this loop, we make a request to the DAS API using helius.rpc.getAssetsByGroup(). We provide the group key, group value, and current page number.
- We process the items in the response. If the number of items is less than 1000, we know we've fetched all items, so we set paginate to false to exit the loop. If not, we increment the page variable to fetch the next set of items in the next loop iteration.
- For each item in the response, we extract the name and image from the metadata, and add it to our results array.
- Finally, we store the results in local storage (so we don't have to fetch them again next time), and return the results.
Here is the full code:
Remember to replace 'YOUR_API_KEY' with your actual Helius API key and groupValue with the actual value of the collection you want to display. In this example, our groupValue is 8Rt3Ayqth4DAiPnW9MDFi63TiQJHmohfTWLMQFHi4KZH which is the collection ID for Solana Monkey Business Gen 3.
Step 3: Create the Collection component
- In the $lib/components directory, create a new file named Collection.svelte. Inside this file, set up the necessary imports in the <script> tag.
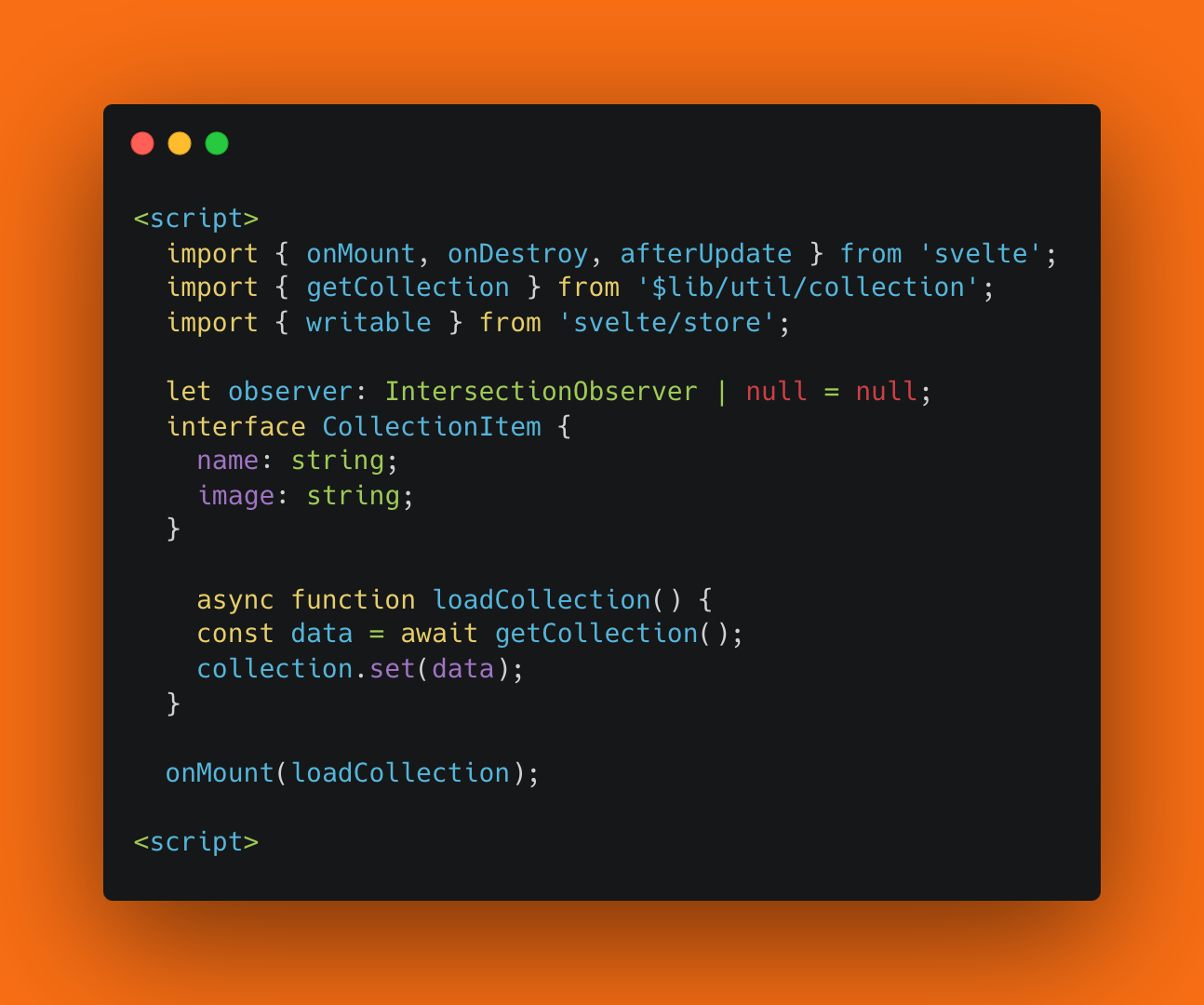
In this code we are:
- Importing our getCollection function to use in this component.
- Importing onMount, onDestroy, and afterUpdate from svelte.
- Setting our collection to an empty type to receive a name and image for each NFT.
- Setting up our onMount function to call the getCollection function, then set our collection data.
- Now we can set our Intersection Observer to only render images when they come into view. This is especially important when you are loading as many images as we are.
.png)
- Now we can set up our onDestroy to stop the observer we set up in the previous afterUpdate function.
.png)
- Now we can set up our grid with tailwind to be in a grid format, and be responsive on all screen sizes:
.png)
- Set up the interior of our grid to load images from the return into the display:
.png)
In the above code we are:
- Using the outer div to set the grid and columns using tailwind.
- Place an each block here to load the image source for each item.
- Adding a card and image div to the grid layout for each NFT item returned to display.
Here is the full code:
.png)
Step 4: Create the page to display the collection
- In your src/routes directory, create a new file named +page.svelte and input the following code:
.png)
In this step we are importing our collection component into our main page.
- Next, set up the layout for the page.
.png)
In this step we are:
- Setting our container for our page content to be placed in.
- Adding a header (styled in the <style>) tag.
- Placing our imported Collection.svelte right under the <h1> in the container.
Step 5: Start the server
Now you are ready to start your SvelteKit server. Run the following command in your terminal:
Conclusion
Congratulations! You have successfully set up a SvelteKit project that uses the Helius SDK to fetch and display a collection of NFTs. The function set up in collection.ts is flexible and can be utilized in different frameworks. This tutorial sets the groundwork for leveraging the DAS SDK for more complex use cases.